Converting Pine Script to MQL5: A Comprehensive Guide
Are you looking to migrate your TradingView trading strategies to MetaTrader 5? Converting Pine Script to MQL5 can be a game-changer for traders who want to leverage the power of both platforms. In this guide, I’ll walk you through the essential steps and considerations for a successful conversion process.
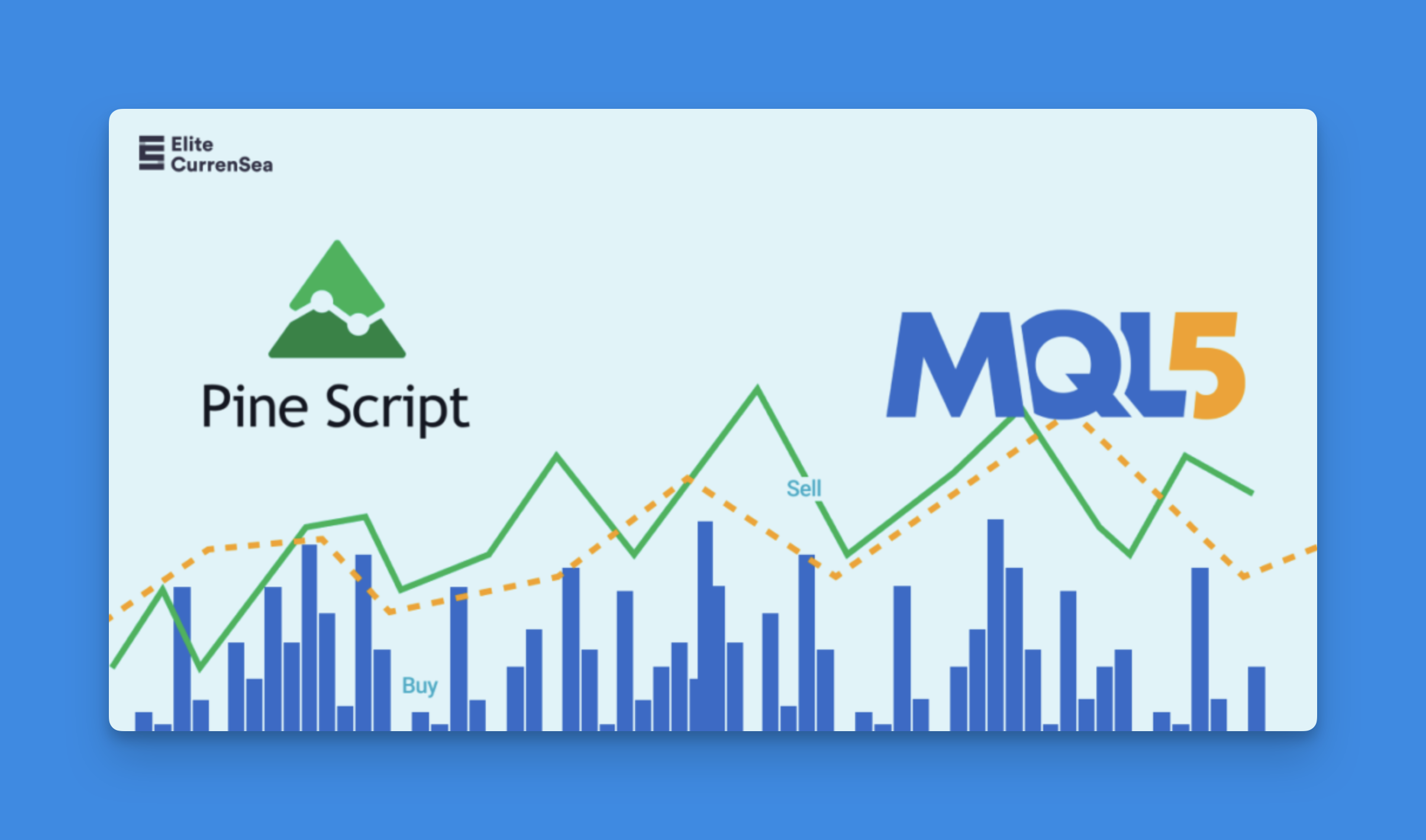
Understanding the Basics
Pine Script and MQL5 are programming languages designed for different trading platforms. Pine Script is TradingView’s proprietary language, while MQL5 is used in MetaTrader 5. Though they serve similar purposes, their syntax and functionality differ significantly.
The conversion process requires understanding both languages’ fundamentals and mapping Pine Script functions to their MQL5 equivalents. This knowledge ensures that your trading strategy maintains its original logic and effectiveness after conversion.
Key Differences Between Pine Script and MQL5
Before diving into the conversion process, it’s important to understand the main differences between these languages:
- Syntax Structure: Pine Script has a simpler, more concise syntax compared to MQL5’s more complex, C++-like structure
- Function Equivalents: Many Pine Script built-in functions have different names and parameters in MQL5
- Variable Declaration: MQL5 requires explicit variable type declaration, unlike Pine Script’s more flexible approach
- Plotting and Visualization: The methods for displaying indicators and visual elements differ significantly

Understanding Pine Script Structure Through Visual Editors
When converting Pine Script to MQL5, one of the greatest challenges is understanding the underlying structure and logic of your original indicators. Visual editors like Pineify provide valuable insights into how Pine Script indicators function, making the conversion process more straightforward.
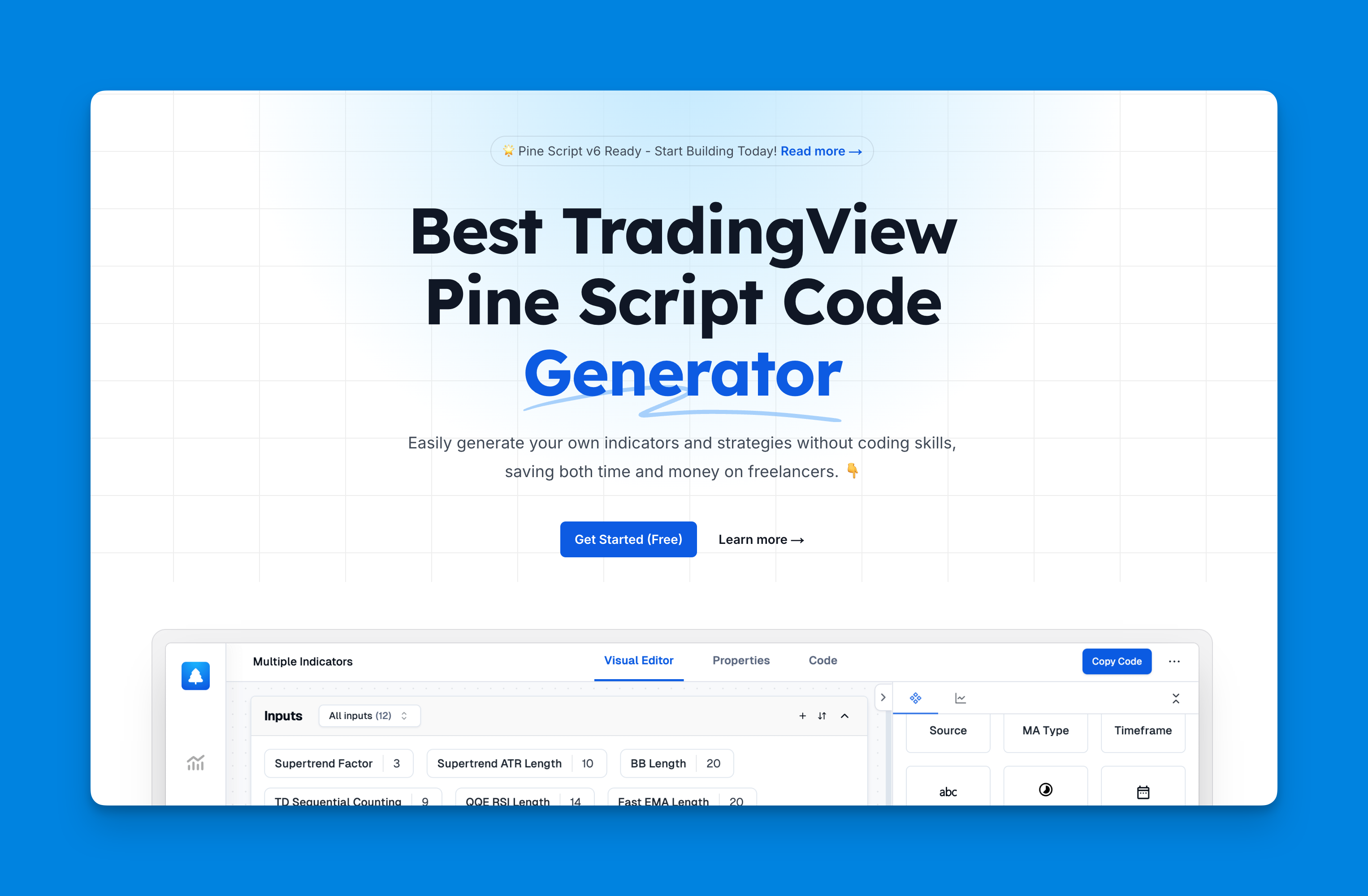
Pineify allows traders to create and manage trading indicators and strategies without programming knowledge, which can help you deconstruct complex indicators into their fundamental components1. By using a visual approach to Pine Script, you can more easily identify the core technical indicators, conditions, and plotting mechanisms that you'll need to recreate in MQL5.
Website: Pineify
Click here to view all the features of Pineify.Step-by-Step Conversion Process
1. Analyze the Original Pine Script
Begin by thoroughly reviewing your Pine Script code. Identify key components such as:
- Input parameters and their default values
- Indicators and calculation methods
- Entry and exit conditions
- Plotting and visualization elements
Understanding the original code’s structure and functionality is crucial for accurate conversion.
2. Map Pine Script Functions to MQL5 Equivalents
Create a mapping of Pine Script functions to their MQL5 counterparts. For example:
- Pine Script’s
sma(close, length)
becomesiMA(NULL, 0, length, 0, MODE_SMA, PRICE_CLOSE, 0)
in MQL5 - Pine Script’s
ema(close, length)
becomesiMA(NULL, 0, length, 0, MODE_EMA, PRICE_CLOSE, 0)
in MQL5
This mapping will serve as your reference throughout the conversion process.
3. Structure Your MQL5 Code
MQL5 requires a specific structure for indicators or expert advisors. A basic template includes:
// Include necessary libraries
#include <Trade\Trade.mqh>
// Input parameters
input int Length = 14; // Default value from Pine Script
// Global variables
double MovingAverage[];
// Initialization function
int OnInit()
{
// Setup code here
return(INIT_SUCCEEDED);
}
// Main calculation function
void OnTick()
{
// Main strategy logic here
}
4. Implement the Logic
Translate your Pine Script logic into MQL5, ensuring that calculations and conditions remain the same. Pay special attention to:
- Order execution methods
- Risk management functions
- Indicator calculations
- Entry and exit conditions
5. Test and Optimize
After conversion, thoroughly test your MQL5 code to ensure it behaves as expected. Compare results with the original Pine Script strategy to identify any discrepancies.
Common Challenges and Solutions
Handling Pine Script Libraries
If your Pine Script uses custom libraries, you’ll need to recreate these functions in MQL5. Consider creating separate include files for complex functions to maintain code organization.
Adapting to MQL5’s Event-Driven Model
Unlike Pine Script’s linear execution, MQL5 uses an event-driven model. Your code needs to respond to specific events like OnTick()
, OnCalculate()
, or OnTimer()
.
Managing Memory Efficiently
MQL5 requires more explicit memory management. Use dynamic arrays appropriately and ensure proper initialization to avoid memory leaks.
Tools to Assist Conversion
Several tools can help streamline the conversion process:
- AI-powered code converters specifically designed for Pine Script to MQL5 translation
- Documentation resources that provide function equivalents between the two languages
- Community forums where experienced programmers share conversion tips and solutions
Real-World Example
Let’s look at a simple moving average crossover strategy conversion:
Pine Script:
//@version=4
strategy("Simple MA Crossover")
fast_length = input(9, title="Fast Length")
slow_length = input(21, title="Slow Length")
fast_ma = sma(close, fast_length)
slow_ma = sma(close, slow_length)
if (crossover(fast_ma, slow_ma))
strategy.entry("Buy", strategy.long)
if (crossunder(fast_ma, slow_ma))
strategy.entry("Sell", strategy.short)
plot(fast_ma, color=color.blue)
plot(slow_ma, color=color.red)
MQL5 Equivalent:
#include <Trade\Trade.mqh>
input int FastLength = 9; // Fast Length
input int SlowLength = 21; // Slow Length
CTrade trade;
double FastMA[], SlowMA[];
int OnInit()
{
ArraySetAsSeries(FastMA, true);
ArraySetAsSeries(SlowMA, true);
return(INIT_SUCCEEDED);
}
void OnTick()
{
// Calculate MAs
int copied1 = CopyBuffer(iMA(_Symbol, _Period, FastLength, 0, MODE_SMA, PRICE_CLOSE), 0, 0, 3, FastMA);
int copied2 = CopyBuffer(iMA(_Symbol, _Period, SlowLength, 0, MODE_SMA, PRICE_CLOSE), 0, 0, 3, SlowMA);
if(copied1 <= 0 || copied2 <= 0) return;
// Check for crossover (Buy)
if(FastMA[1] <= SlowMA[1] && FastMA[0] > SlowMA[0])
{
trade.Buy(0.1);
}
// Check for crossunder (Sell)
if(FastMA[1] >= SlowMA[1] && FastMA[0] < SlowMA[0])
{
trade.Sell(0.1);
}
}
Final Considerations
When converting Pine Script to MQL5, remember that the goal is not just to translate code but to preserve the strategy’s effectiveness. Take time to understand both languages’ nuances and test thoroughly after conversion.
For complex strategies, consider hiring a professional programmer experienced in both languages to ensure accurate conversion.