Essential Pine Script v6 Cheat Sheet for Traders
Overviewβ
This cheat sheet provides a comprehensive guide to Pine Script V6, a powerful scripting language used in TradingView for creating custom technical analysis tools. It covers essential operators, keywords, storage methods, built-in types, user-defined types, variables, constants, and built-in functions. Additionally, it introduces Pineify, a tool that simplifies Pine Script generation for traders without coding knowledge.
Key Sections:β
- Operators: Basic math, comparison, logic, and assignment operators.
- Keywords: Reserved words and their purposes.
- Storage Methods: Different ways to store and organize data.
- Built-in Types: Fundamental data types in Pine Script.
- User-Defined Types: Creating custom data structures.
- Variables and Constants: Understanding variable types and assignments.
- Built-in Functions: Commonly used functions categorized by their purposes.
- Notes on Pine Script: Key concepts and best practices.
- Tips for Writing Pine Script: Practical advice for effective scripting.
- Generating Pine Script with Pineify: Using Pineify to create scripts without coding.
This guide aims to make Pine Script accessible to both beginners and experienced programmers, providing clear explanations and practical examples.
Operatorsβ
Basic Math Operatorsβ
These operators help you perform everyday calculations in your trading scripts:
Operator | Purpose |
---|---|
+ | Adds two numbers together |
- | Subtracts one number from another |
* | Multiplies two numbers |
/ | Divides one number by another |
% | Shows what's left over after division |
Comparison Operatorsβ
Operator | Purpose |
---|---|
== | Checks if two values are exactly the same |
!= | Checks if two values are different |
> | Checks if the first value is bigger |
\ | Checks if the first value is smaller |
>= | Checks if the first value is bigger or the same |
<= | Checks if the first value is smaller or the same |
Logic Operatorsβ
These operators help you combine or check conditions:
Operator | Purpose |
---|---|
and | Both conditions must be true |
or | At least one condition must be true |
not | Reverses a condition (true becomes false, false becomes true) |
?: | A shortcut for if-then-else decisions |
Assignment Operatorsβ
These operators help you store and update values:
Operator | Purpose |
---|---|
= | Gives a value to a variable |
:= | Changes a variable's value |
+= | Adds to the current value |
-= | Subtracts from the current value |
*= | Multiplies the current value |
/= | Divides the current value |
%= | Updates with the division remainder |
Keywordsβ
Basic Keywordsβ
These are special words in Pine Script that you can't use as variable names:
Keyword | Simple Explanation |
---|---|
import | Brings in useful functions from other scripts, like borrowing a tool from a friend |
export | Makes your functions available for other scripts to use, like sharing your tools |
method | Creates a special function that belongs to a custom data type |
type | Makes a custom container for holding related data together |
matrix | Helps work with data arranged in rows and columns, like a spreadsheet |
var | Creates a box to store information that stays the same until you change it |
varip | Like 'var', but updates more frequently within the same price bar |
Words You Can't Useβ
Pine Script has some reserved words that you should avoid using as names in your code:
Keyword | Purpose |
---|---|
catch | Used for handling errors |
class | For organizing code |
do | For repeating actions |
ellipse | For drawing oval shapes |
in | For checking if something is part of a group |
is | For comparing things |
polygon | For drawing shapes with multiple sides |
range | For working with number ranges |
return | For sending back results from functions |
struct | For grouping related data |
text | For working with words and sentences |
throw | For reporting errors |
try | For testing code that might have problems |
Think of these keywords as the building blocks of Pine Script - they're like special tools that help you create trading strategies and indicators.
Storage Methodsβ
In Pine Script, there are different ways to store and organize data. Hereβs a simple breakdown of these storage methods using "string" as an example:
Storage Type | Description |
---|---|
Matrix | A table with rows and columns, like a spreadsheet. It helps organize data in two dimensions. |
Array | A list where items are arranged in a specific order. This is a one-dimensional structure that holds data in sequence. |
String[] | An older way to describe an array specifically for text data. |
String | A single piece of text or data point. |
These methods help you decide how to structure your data when writing scripts in Pine Script.
Built-in Typesβ
Pine Script provides several basic data types that you can use to create trading indicators and strategies. Here's a simplified explanation of these types:
Type | Description | Example |
---|---|---|
String | Used for text data | "Hello, World!" |
Integer (int) | Whole numbers | 1, 2, 100 |
Float | Numbers with decimals | 1.5, 3.14 |
Boolean (bool) | True/false values | true, false |
Color | Color definitions | RGB values |
Line | Drawable chart lines | Trend lines |
Linefill | Area between lines | Filled regions |
Box | Rectangular shapes | Chart boxes |
Label | Text annotations | Chart labels |
Table | Data organization | Visual data tables |
These data types are the building blocks of Pine Script, allowing you to handle various kinds of information and display it effectively in your trading tools.
User-Defined Typesβ
Here's a simplified explanation of User-Defined Types in Pine Script, presented in a clear, tabular format:
Component | Description | Example |
---|---|---|
Basic Structure | A custom data type you create to group related data together | Similar to creating a template for organizing related information |
Creation Keyword | Use the type keyword to create a new type | type MyCustomType |
Fields | Variables inside the type that store different kinds of data | Like properties that describe your custom type |
Field Types | Can be simple (numbers, text) or complex (arrays, matrices) | float price = 0.0 for a simple type |
Default Values | Only allowed for simple types (numbers, text, true/false) | Cannot set defaults for arrays or matrices |
Instance Creation | Use .new() to create a new copy of your type | MyCustomType.new() |
Field Access | Use a dot (.) to access fields of your type | myInstance.price |
Example Structureβ
type TradeSetup
float entryPrice = 0.0 // Allowed default
string direction // Allowed default
array signals // Not allowed default
This structure helps organize trading-related data into a single, manageable unit. The type system ensures that your data stays organized and consistent throughout your trading script.
Variables and Constantsβ
Here is a simplified and accessible table summarizing the "Variables and Constants" section from the Pine Script 5 Mini Reference:
Concept | Explanation |
---|---|
Loosely Typed | You don't need to specify the type of a variable when you create it. |
Type Inference | The type of a variable is determined by the value you assign to it. |
Immutable Type | Once a variable has a type, you cannot change it to another type later. |
Examples of Variables in Pine Scriptβ
Variable | Assigned Value | Inferred Type |
---|---|---|
a | 1 | Integer |
b | 1.2 | Float |
c | "1.2" | String |
d | true | Boolean |
e | color.new(color.red, 50) | Color |
This table provides an easy-to-understand overview of how variables and constants work in Pine Script, making it accessible for users without technical backgrounds.
Built-in Functionsβ
The Pine Script built-in functions can be organized into clear categories for easier understanding. Here's a simplified overview of the most commonly used functions:
Technical Analysis Functionsβ
What You Want to Do | Function to Use | Simple Explanation |
---|---|---|
Track Money Flow | ta.mfi() | Measures if money is flowing in or out of an asset |
Measure Momentum | ta.rsi() | Shows if price movement is getting stronger or weaker |
Find Price Averages | ta.sma() , ta.ema() | Calculates average prices over time |
Spot Price Channels | ta.bb() , ta.kc() | Shows price ranges where trading typically happens |
Track Volume Patterns | ta.obv , ta.pvt | Helps understand if volume supports price moves |
Math Helper Functionsβ
What You Want to Do | Function to Use | Simple Explanation |
---|---|---|
Basic Math | math.abs() , math.round() | Handle common math operations |
Advanced Calculations | math.log() , math.sqrt() | Perform complex mathematical operations |
Get Random Numbers | math.random() | Generate random values |
Find Highest/Lowest | math.max() , math.min() | Find extreme values in a set |
Time and Data Functionsβ
What You Want to Do | Function to Use | Simple Explanation |
---|---|---|
Work with Dates | time.year , time.month | Get different parts of dates |
Handle Time Zones | time.timezone | Work with different time zones |
Get Market Data | request.security() | Fetch data from other markets |
Get Company Info | request.financial() | Access company financial data |
Array Functionsβ
What You Want to Do | Function to Use | Simple Explanation |
---|---|---|
Create Lists | array.new() | Make a new list of items |
Change Lists | array.push() , array.pop() | Add or remove items from lists |
Find Items | array.indexof() | Locate items in a list |
Sort Data | array.sort() | Arrange items in order |
Matrix Functionsβ
What You Want to Do | Function to Use | Simple Explanation |
---|---|---|
Create Tables | matrix.new | Make a new data table |
Change Data | matrix.set , matrix.get | Add or retrieve values |
Analyze Tables | matrix.sum , matrix.avg | Calculate table statistics |
Transform Tables | matrix.transpose | Reorganize table data |
Notes on Pine Scriptβ
Here's a simplified guide to Pine Script's key concepts presented in an easy-to-understand table format:
Concept | What It Is | What You Need to Know |
---|---|---|
Storage Methods | Ways to organize data | β’ Simple Type: Store single values like numbers or text β’ Array: Store lists of values β’ Matrix: Store data in rows and columns |
User Defined Types (UDTs) | Custom data containers you create | β’ Like creating your own recipe for organizing data β’ Must have at least one field β’ Can only use simple values as defaults β’ Names can't start with numbers |
Functions | Reusable blocks of code | β’ Must have a name and at least one input (parameter) β’ Can return values β’ Can have default values for inputs β’ Can be shared with other scripts if marked as "export" |
Comments | Notes in your code | β’ Start with "//" β’ Help explain what your code does β’ Run until the end of the line |
Documentation Tags | Special notes that explain code | β’ Start with "//@" β’ Describe functions, types, and fields β’ Help others understand your code β’ Can use multiple lines |
Default Values | Pre-set values for inputs | β’ Work with simple types (numbers, text, true/false) β’ Can't use complex types (arrays, matrices) β’ Must appear at the end of parameter lists |
Tips for Writing Pine Scriptβ
- Keep names simple and descriptive β’ Use comments to explain complex logic β’ Start with basic types before using advanced features β’ Test your code with simple examples first
This reference aims to help both beginners and experienced programmers understand Pine Script's core concepts without getting lost in technical jargon.
Generating Pine Script with Pineifyβ
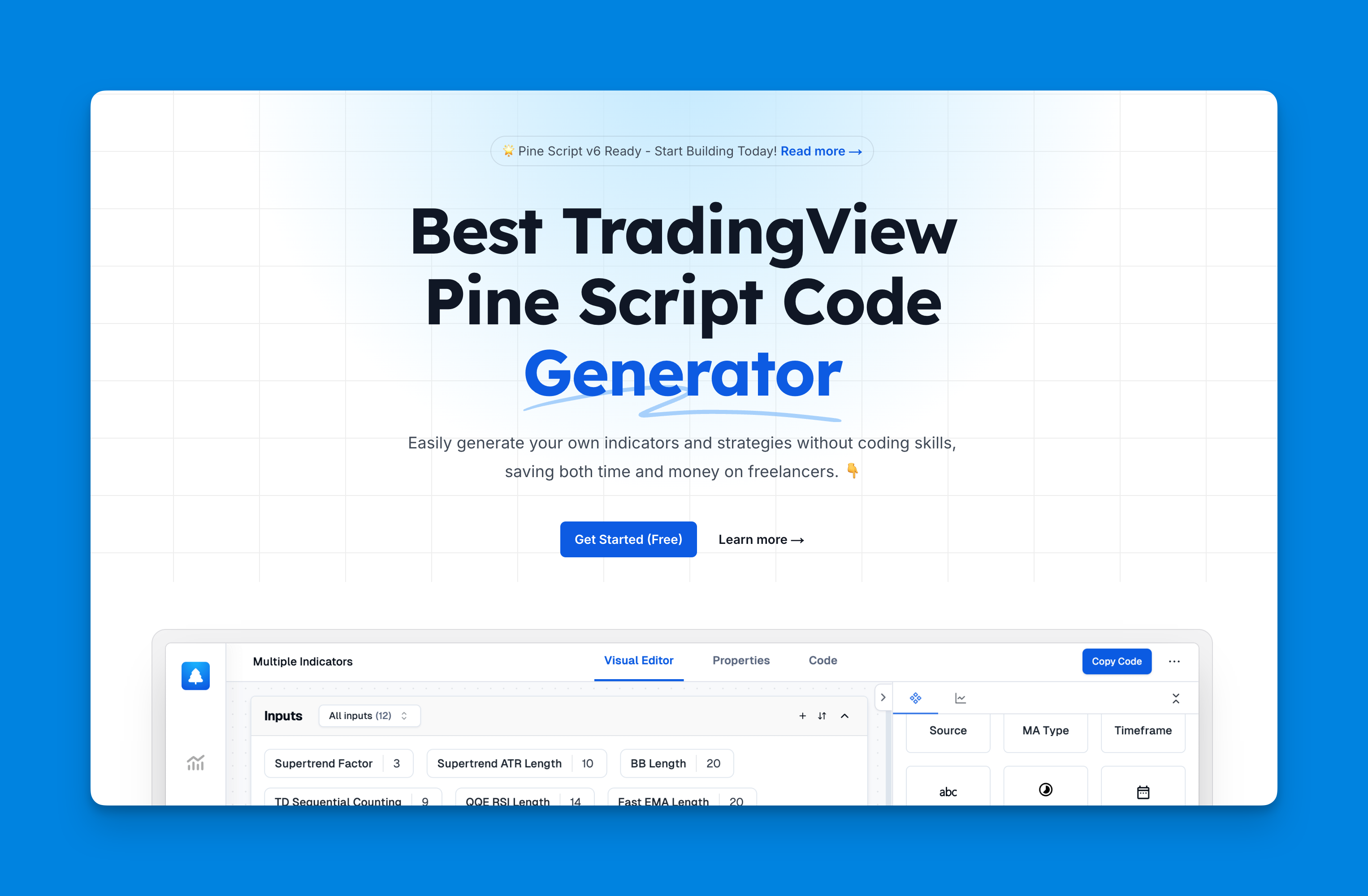
Website: Pineify
One of the most exciting developments in the world of trading is the ability to create custom scripts without needing any coding knowledge. Pineify is a tool that makes this possible by allowing users to generate Pine Script effortlessly. Whether you're a seasoned trader or a beginner, Pineify can simplify your script creation process.
Click here to view all the features of Pineify.What is Pineify?β
Pineify is an intuitive platform designed to help traders create Pine Scripts for TradingView without writing a single line of code. It uses a user-friendly interface where you can define your trading strategies and indicators through simple selections and inputs.
How Does It Work?β
- User-Friendly Interface: Pineify provides a graphical interface where you can select from a variety of pre-defined options and parameters. This means you can focus on the logic and strategy rather than syntax and coding details.
- Customization: Despite its simplicity, Pineify offers extensive customization options. You can adjust parameters, set conditions, and even combine multiple indicators to tailor the script to your specific needs.
- Instant Script Generation: Once you've set up your strategy, Pineify generates the corresponding Pine Script code instantly. You can then copy this code directly into TradingView to test and implement your strategy.
Benefits of Using Pineifyβ
- No Coding Required: Perfect for those who want to leverage TradingView's powerful scripting capabilities without learning the intricacies of coding.
- Time-Saving: Quickly create and test different strategies without spending hours writing and debugging code.
- Accessibility: Opens up advanced trading tools to a wider audience, making it easier for anyone to develop and refine their trading strategies.
Getting Started with Pineifyβ
To start generating scripts with Pineify, simply visit their website, sign up for an account, and explore the available features. The platform often includes tutorials and guides to help you get the most out of its capabilities.
By using tools like Pineify, traders can focus on developing effective strategies while leaving the technical aspects of coding behind. This democratizes access to powerful trading tools, making them accessible to everyone, regardless of their technical background.