EMA Pine Script: Customization for Effective Trading
The Exponential Moving Average (EMA) is a vital tool in the arsenal of traders, providing insights into price trends and potential market reversals. This article delves into the intricacies of EMA in Pine Script, a powerful scripting language used on TradingView. We will explore how to implement EMAs, create custom indicators, and develop trading strategies.

Understanding the Exponential Moving Average (EMA)

The Exponential Moving Average (EMA) is a type of moving average that places greater weight on the most recent data points. Unlike the Simple Moving Average (SMA), which treats all data points equally, the EMA reacts more quickly to price changes, making it a preferred choice for many traders.
Key Characteristics of EMA
- Sensitivity: EMAs are more responsive to recent price changes compared to SMAs.
- Trend Identification: Traders use EMAs to identify bullish or bearish trends.
- Signal Generation: Crossovers between different EMAs can signal potential buy or sell opportunities.
Getting Started with Pine Script
Pine Script is a domain-specific language designed for creating custom technical indicators and strategies on TradingView. To begin coding with Pine Script, you need access to the Pine Editor within TradingView.
Writing Your First EMA Script
To calculate an EMA in Pine Script, you can use the built-in ta.ema
function. Here’s a basic example:
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("[Pineify - Best Pine Script Generator] Simple EMA", overlay=true)
length = input(14, title="EMA Length")
emaValue = ta.ema(close, length)
plot(emaValue, color=color.blue, title="EMA")
In this script:
- The
input
function allows users to specify the EMA length. - The
ta.ema
function calculates the EMA based on closing prices. - The
plot
function displays the EMA on the chart.
Add EMA Indicator on Pineify
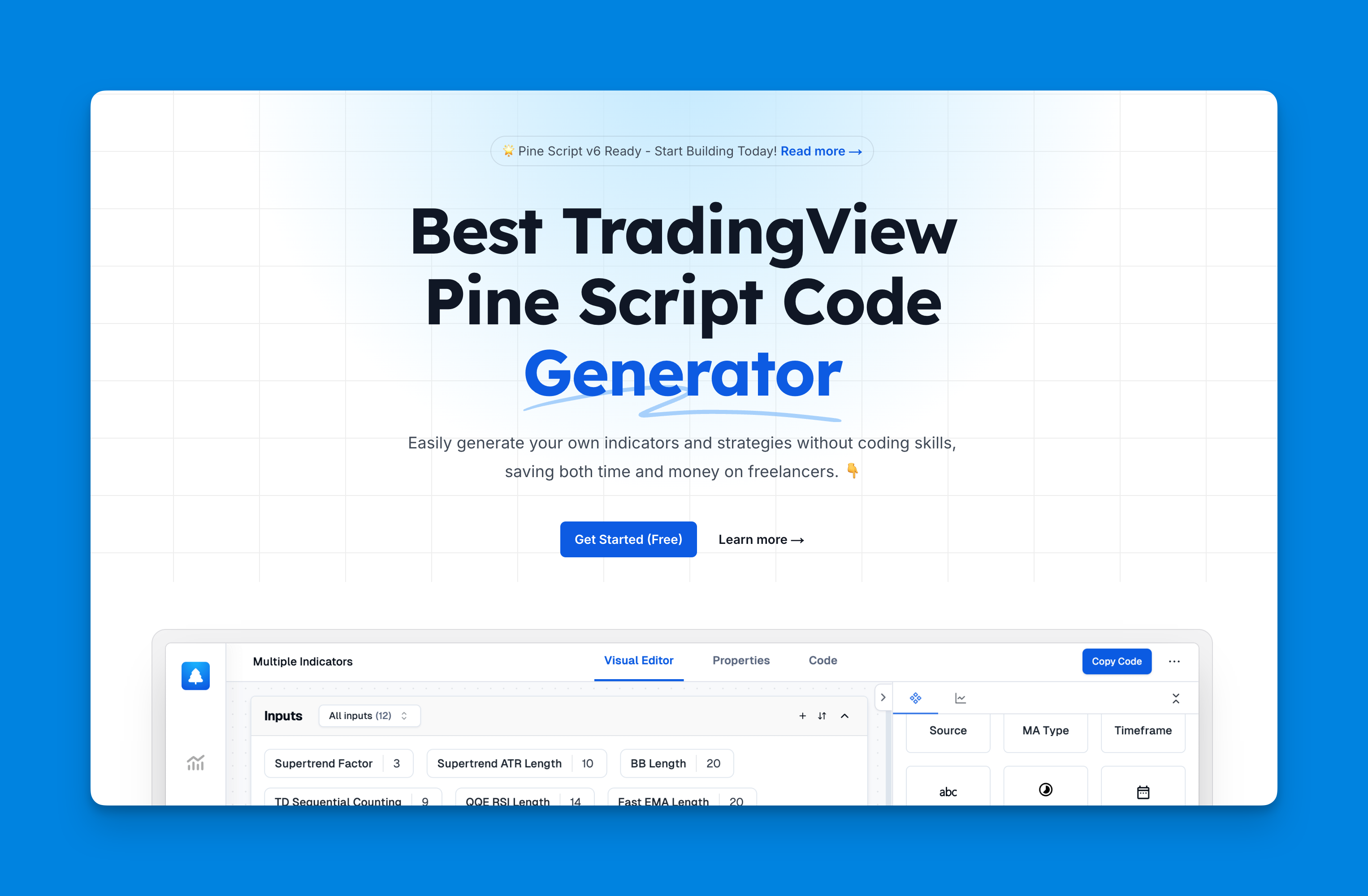
Website: Pineify
To enhance your trading experience with the Exponential Moving Average (EMA) indicator, consider utilizing Pineify, a powerful tool designed for traders using TradingView.
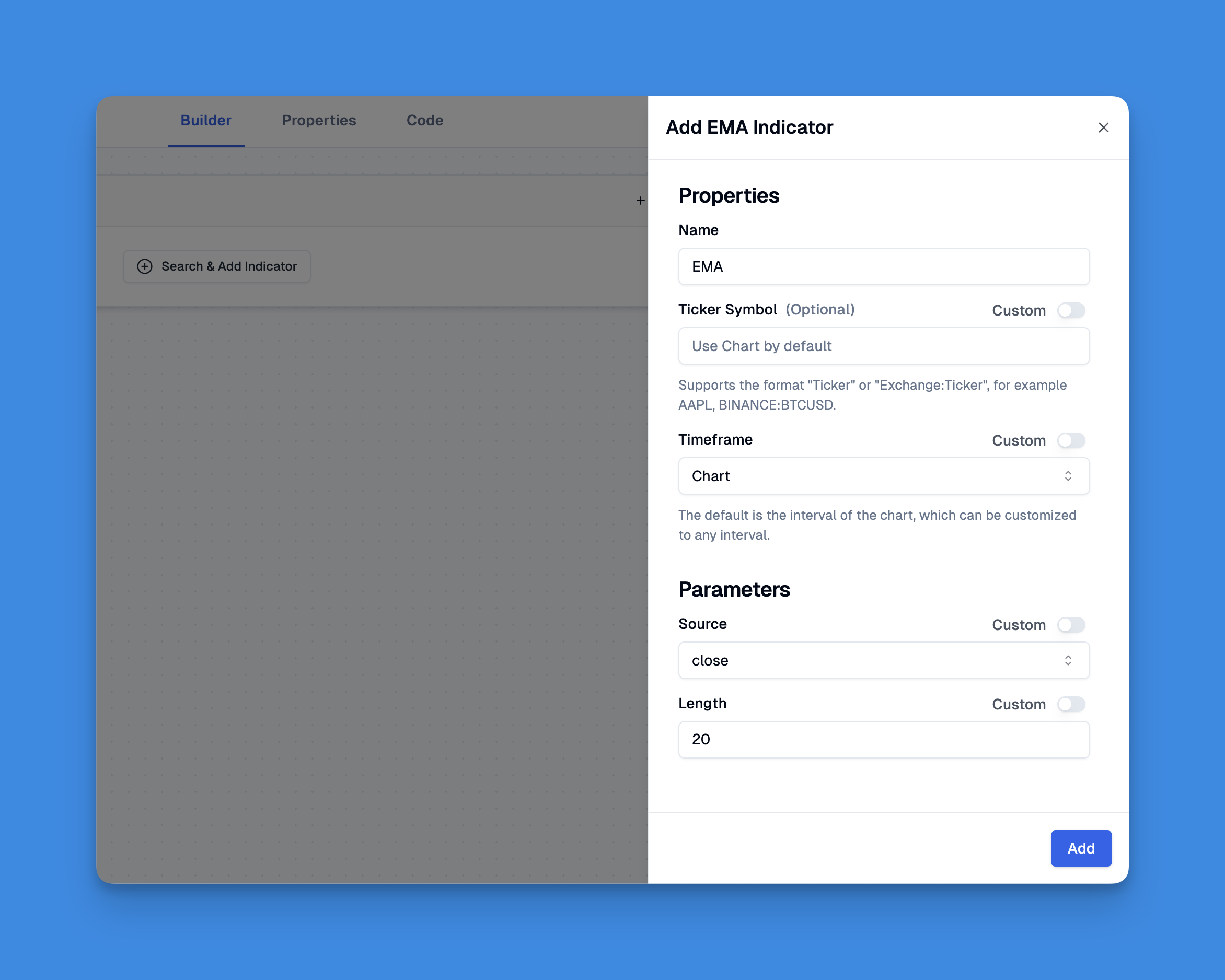
With Pineify's intuitive interface, you can quickly generate and customize your EMA indicator to suit your specific trading strategy. The platform supports various ticker symbols and timeframes, allowing you to tailor your analysis to different market conditions. Pineify allows you to add unlimited technical indicators to your charts, effectively bypassing TradingView's restrictions on the number of indicators you can use simultaneously.
Click here to view all the features of Pineify.
Customizing Your EMA Indicator
Customization enhances user experience and provides flexibility in trading strategies. You can allow users to adjust parameters such as color and line style.
Example of a Customizable EMA Script
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("[Pineify - Best Pine Script Generator] Customizable EMA", overlay=true)
length = input(14, title="EMA Length")
colorChoice = input(color.blue, title="Line Color")
emaValue = ta.ema(close, length)
plot(emaValue, color=colorChoice, title="EMA")
Implementing Multiple EMAs
Traders often use multiple EMAs to identify trends and potential reversals. Here’s how you can implement multiple EMAs in one script:
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("[Pineify - Best Pine Script Generator] Multiple EMAs", overlay=true)
shortLength = input(9, title="Short EMA Length")
longLength = input(21, title="Long EMA Length")
shortEma = ta.ema(close, shortLength)
longEma = ta.ema(close, longLength)
plot(shortEma, color=color.red, title="Short EMA")
plot(longEma, color=color.green, title="Long EMA")
Creating an EMA Crossover Strategy
An effective trading strategy often involves using crossovers between different EMAs. Here’s an example of a simple crossover strategy:
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
strategy("[Pineify - Best Pine Script Generator] EMA Crossover Strategy", overlay=true)
shortEma = ta.ema(close, 9)
longEma = ta.ema(close, 21)
plot(shortEma, color=color.red)
plot(longEma, color=color.green)
if (ta.crossover(shortEma, longEma))
strategy.entry("Long", strategy.long)
if (ta.crossunder(shortEma, longEma))
strategy.entry("Short", strategy.short)
In this script:
- Crossover Logic: The strategy enters a long position when the short EMA crosses above the long EMA and vice versa for short positions.
- Visual Representation: Both EMAs are plotted for easy visualization.
Utilizing Higher Timeframe EMAs
Accessing higher timeframe data can provide additional insights into market trends. You can use the security()
function to reference higher timeframes in your script.
Example of Higher Timeframe EMA
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("[Pineify - Best Pine Script Generator] Higher Timeframe EMA", overlay=true)
length = input(50, title="EMA Length")
res = input.timeframe("D", title="Higher Timeframe")
higherTimeframeEma = request.security(syminfo.tickerid, res, ta.ema(close, length))
plot(higherTimeframeEma, color=color.orange)
Best Practices for Writing Pine Scripts
- Comment Your Code: Use comments to explain complex logic or functions within your script.
- Test Thoroughly: Always backtest your strategies using TradingView’s built-in tools before applying them in live trading.
- Engage with Community: Share your scripts with the TradingView community for feedback and improvements.
Conclusion
The Exponential Moving Average is a powerful tool that can greatly enhance your trading strategies when implemented correctly in Pine Script. By understanding how to create and customize your own scripts on TradingView, you can tailor your trading experience to suit your specific needs.
References
- https://www.tradingview.com/scripts/ema/
- https://zenandtheartoftrading.com/pinescript/higher-timeframe-ema/
- https://www.tradingview.com/pine-script-docs/v4/quickstart-guide/
- https://pinewizards.com/technical-analysis-functions/ta-ema-function-in-pine-script/
- https://www.youtube.com/watch?v=1DcOxF-VedA
- https://stackoverflow.com/questions/78436502/pinescript-conundrum-ema-crossover-strategy
- https://www.youtube.com/watch?v=awxlkS4Afuk
- https://www.youtube.com/watch?v=wA_CjkYrB1M
- https://www.tradingview.com/pine-script-docs/primer/first-indicator/
- https://gist.github.com/heathdrobertson/4960d17fe9fb256f63d531c2597afd5a
- https://www.youtube.com/watch?v=aYu0oAtwUts