For Loops in Pine Script
Pine Script, TradingView's proprietary language, allows users to create custom trading tools and strategies. Among the essential programming concepts in Pine Script is the for loop, which facilitates the repetition of a block of code for a specified number of times. This article will cover the syntax, applications, and best practices for utilizing for loops in Pine Script to enhance your trading scripts.
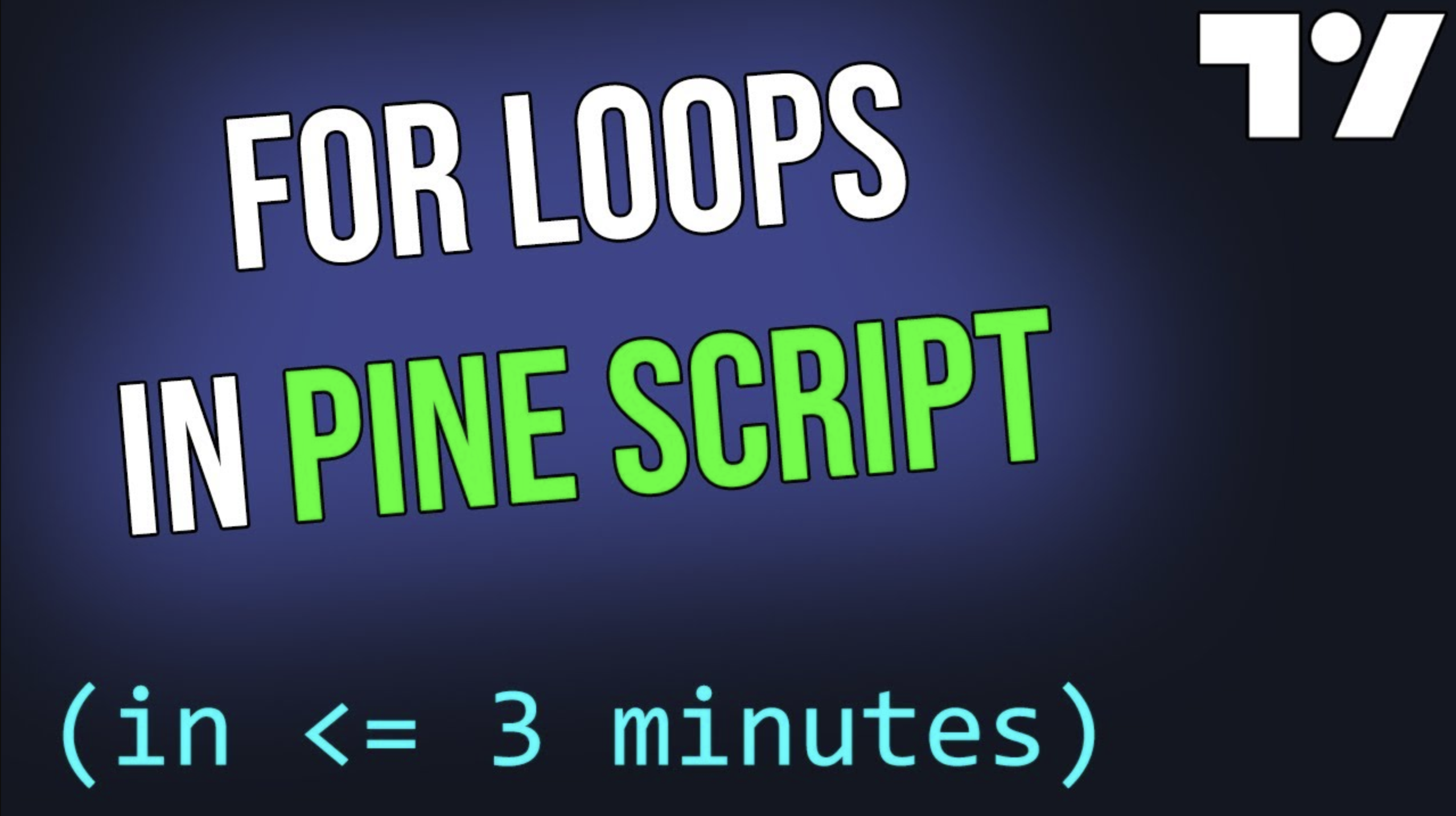
Syntax
For loops are fundamental for performing repetitive tasks, such as calculations and data analysis, within a defined range.
The basic structure of a for loop in Pine Script is:
for counter = start_value to end_value
// Code to be repeated
counter
: A variable that increments with each iteration of the loop.start_value
: The initial value of the counter.end_value
: The maximum value the counter can reach, determining when the loop stops.
How For Loops Work

A for loop executes a block of code repeatedly, incrementing the counter variable after each iteration until it reaches the end value.
Practical Applications
For loops are useful for various tasks in Pine Script:
- Analyzing historical data.
- Managing arrays.
- Implementing complex trading logic.
- Performing iterative calculations.
Generator Pine Script Code Without Coding
Pineify is a free AI Pine Script v6 code generator for TradingView scripts that allows users to easily generate their own indicators and strategies without coding skills, saving both time and money on freelancers.
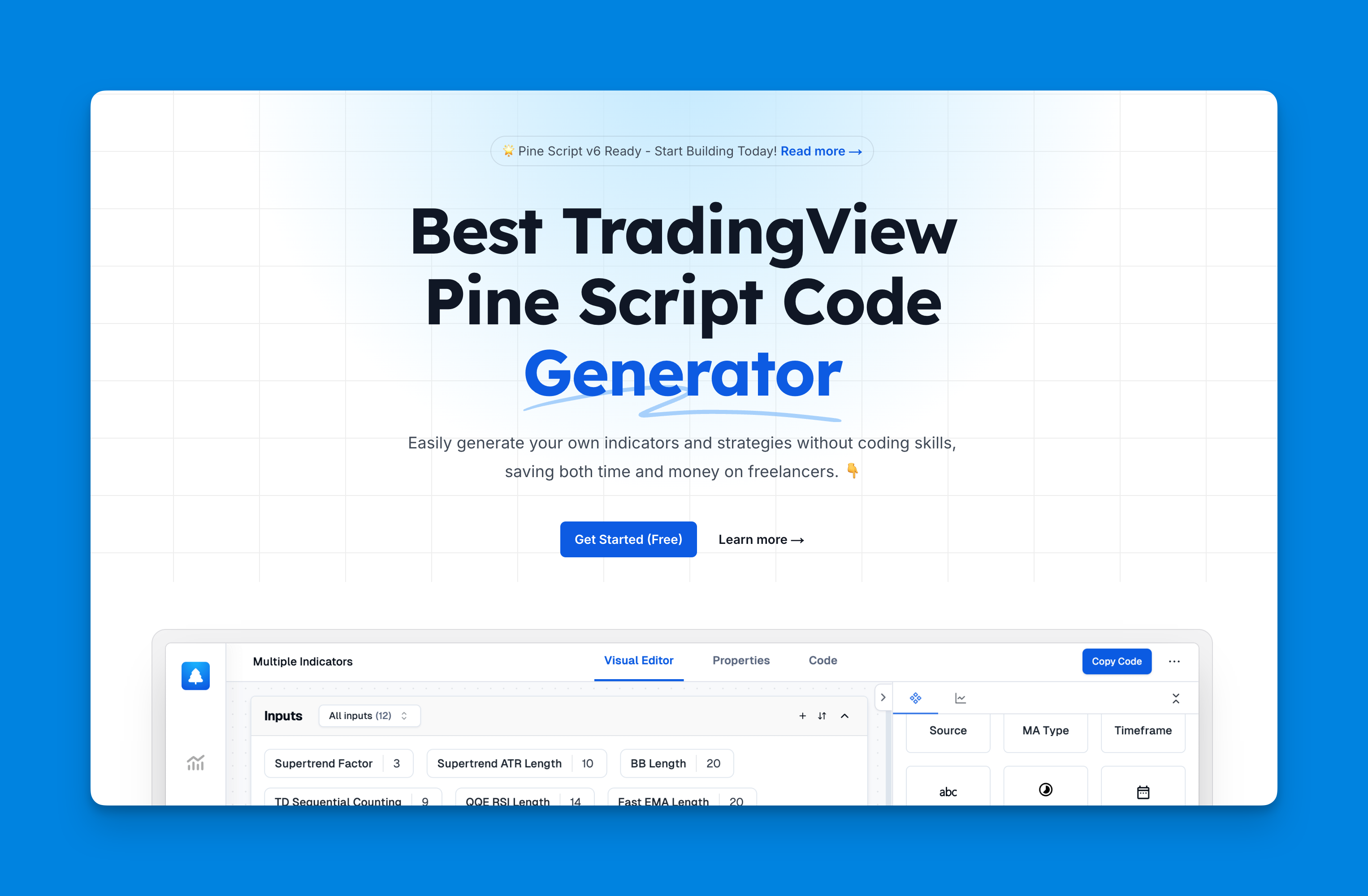
Website: Pineify
Key Features and Benefits:
- No Coding Required Create and customize indicators and strategies easily without any programming knowledge.
- Unlimited Indicators Add more than two indicators to your charts with just the TradingView free plan.
- Import Custom Code Easily import and customize existing Pine Script indicators and strategies.
- Condition Editor Flexibly combine multiple technical indicators, price, and various price data to construct precise and reliable trading rules.
- Strategy Tester Easily set up market orders for immediate trades, while also flexibly using take-profit and stop-loss orders to manage risk and secure profits.
- Time and Cost Savings Generate scripts in minutes and save money compared to hiring a freelancer.
Examples of For Loops
For loops can be used to perform calculations and create visualizations. However, plots can only work outside local scopes, so you can only see the final loops result using a plot. To overcome this limitation, use labels inside the local scope.
The below examples demonstrate how to use "for" loops in Pine Script for different applications.
Simple Moving Average (SMA)
The math behind the SMA is you loop over the last N bars, add up the series value you want to average from each one, then divide by N.
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("[Pineify - Best Pine Script Generator] SMA with For Loop", overlay=true)
length = input.int(30, title="SMA Length")
src = close
sum = 0.0
for i = 0 to length - 1
sum := sum + src[i]
sma = sum / length
plot(sma, color=color.blue, title="SMA")
In this example:
- The script calculates the SMA of the closing prices over a specified length.
- The for loop iterates through the last 'length' bars, summing their closing prices.
- The SMA is then calculated by dividing the sum by the length.
Counting High and Low Bars
This example counts the number of bars with a high greater or lower than the current bar’s high over a user-defined lookback period.
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("`[Pineify - Best Pine Script Generator] for` loop", overlay=true)
lookbackInput = input.int(50, "Lookback in bars", minval = 1, maxval = 4999)
higherBars = 0
lowerBars = 0
if barstate.islast
var label lbl = label.new(na, na, "", style = label.style_label_left)
for i = 1 to lookbackInput
if high[i] > high
higherBars += 1
else if high[i] < high
lowerBars += 1
label.set_xy(lbl, bar_index, high)
label.set_text(lbl, str.tostring(higherBars, "# higher bars\\n") + str.tostring(lowerBars, "# lower bars"))
In this example:
lookbackInput
: Defines the number of bars to look back.for i = 1 to lookbackInput
: Loops from 1 to the user-defined lookback period.- Inside the loop, we compare the high of each past bar (
high[i]
) to the bar’s high (high
). Depending on the comparison, we increment eitherhigherBars
orlowerBars
. - Finally, a label is created to display the count of higher and lower bars.
Looping Through Arrays
Arrays are very important for Pine Script. Use the “for/in” statement to iterate through an array.
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator("[Pineify - Best Pine Script Generator] For-In", overlay=true)
arr = array.from(1, 5, 2, 4 , 3)
if barstate.islast
label.new(bar_index, close, str.tostring(arr))
sum = 0
for el in arr
sum += el
if barstate.islast
label.new(bar_index + 5, close, str.tostring(sum))
sum_even = 0
for [ind, el] in arr
if ind % 2 == 1
sum_even += el
if barstate.islast
label.new(bar_index + 10, close, str.tostring(sum_even))
plot(close)
In this example:
- The script defines an array
arr
with some initial values. - It calculates the sum of all elements in the array using a
for/in
loop. - It calculates the sum of elements with odd indices using a
for/in
loop. - The array and the calculated sums are displayed using labels on the chart.
Controlling Loop Execution
Pine Script provides keywords to control the flow of for loops:
break
: Exits the loop prematurely based on a condition.continue
: Skips the current iteration and proceeds to the next.
Here's an example illustrating the use of break
and continue
:
// This source code is subject to the terms of the Mozilla Public License 2.0 at https://mozilla.org/MPL/2.0/
// © Pineify
//======================================================================//
// ____ _ _ __ //
// | _ \(_)_ __ ___(_)/ _|_ _ //
// | |_) | | '_ \ / _ \ | |_| | | | //
// | __/| | | | | __/ | _| |_| | //
// |_| |_|_| |_|\___|_|_| \__, | //
// |___/ //
//======================================================================//
//@version=6
indicator(" [Pineify - Best Pine Script Generator] Loop Control", overlay=false)
length = 10
sum = 0
for i = 1 to length
if i == 3
continue // Skip iteration when i equals 3
if i == 7
break // Exit loop when i equals 7
sum := sum + i
plot(sum, title="Sum")
In this example:
- The loop calculates the sum of numbers from 1 to 10, but skips the iteration when
i
is 3 and exits the loop wheni
is 7.
Best Practices
To write efficient and effective for loops in Pine Script, consider the following:
- Always define the loop's start and end values clearly.
- Avoid infinite loops by ensuring the loop condition will eventually be met.
- Use
break
andcontinue
statements judiciously to optimize loop execution. - Be mindful of the scope of variables used within the loop.
- Ensure that plots only work outside local scopes.
Conclusion
For loops are a powerful tool for automating repetitive tasks and implementing complex logic in Pine Script. Understanding how to use for loops effectively can significantly enhance your ability to create custom trading indicators and strategies on TradingView. By mastering this concept and following best practices, you can unlock new possibilities in your trading scripts and improve your technical analysis.
Next Steps
Ready to take your Pine Script skills to the next level? Explore these resources:
- Best Pine Script Generator.
- TradingView's Pine Script documentation.
- Online tutorials and courses.
- Pine Script communities and forums.
Start experimenting with for loops in your own scripts and discover the endless possibilities for creating custom trading tools.